In order to have a DAL router executing CLI commands received via SMS, the following procedure can be followed:
1. Copy the content of the following python script in a txt file (You can find the script also here: https://www.digi.com/resources/documentation/digidocs/90002399/default.htm#os/python-digidevice-sms-c.htm?TocPath=Applications%257CDevelop%2520Python%2520applications%257CPython%2520modules%257CDigidevice%2520module%257C_____10):
#!/usr/bin/python
# Take an incoming SMS message from a specified phone number and run it as
# a CLI command. Send a reponse SMS to the sender before running the command
import os
import threading
import sys
from digidevice import cli
from digidevice.sms import Callback, send
COND = threading.Condition()
allowed_incoming_phone_number = '2223334444'
def sms_test_callback(sms, info):
if info['content.number'] == allowed_incoming_phone_number:
print(f"SMS message from {info['content.number']} received")
print(sms)
print(info)
#if sms == "Reboot":
# send_sms(dest, 'Reboot message received, rebooting device...')
# response = cli.execute("reboot")
# print (response)
send_sms(dest, 'Message received (' + sms + '). Performing as CLI command...')
response = cli.execute(sms)
if not response:
response = 'OK'
send_sms(dest, 'CLI results: ' + response)
print (response)
COND.acquire()
COND.notify()
COND.release()
def send_sms(destination, msg):
print("sending SMS message", msg)
if len(destination) == 10:
destination = "+1" + destination
send(destination, msg)
if __name__ == '__main__':
if len(sys.argv) > 1:
dest = sys.argv[1]
else:
dest = allowed_incoming_phone_number
my_callback = Callback(sms_test_callback, metadata=True)
#send_sms(dest, 'Ready to receive incoming SMS message')
print("Waiting up to 60 seconds for incoming SMS message")
# acquire the semaphore and wait until a callback occurs
COND.acquire()
try:
COND.wait(60.0)
except Exception as err:
print("exception occured while waiting")
print(err)
COND.release()
my_callback.unregister_callback()
os.system('rm -f /var/run/sms/scripts/*') # remove all stored SMS messages, since we've processed them
print("SMS script finished. Please re-run if you want to check for more incoming SMS messages")
os._exit(0)
2. Edit the "allowed_incoming_phone_number" with the number you will use to send CLI via SMS
3. Name the file as "python_sms_to_cli-commands.py"
4. Upload that script onto the device: WEB UI: System>File System > etc > config > scripts (note: to enter in each subfolder, you need to select the main folder and click on the arrow highlighted below):
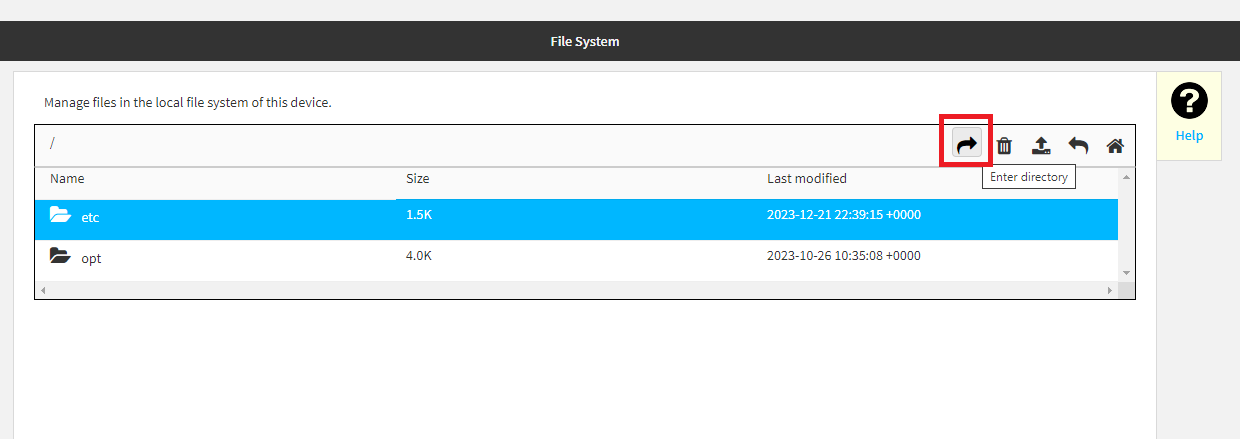
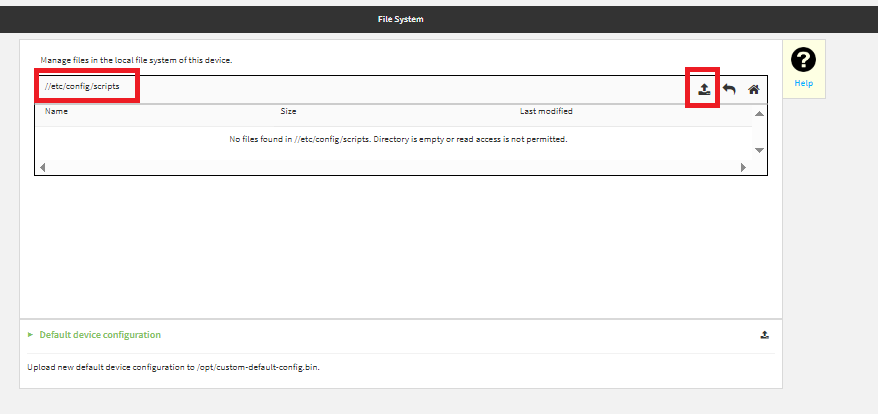
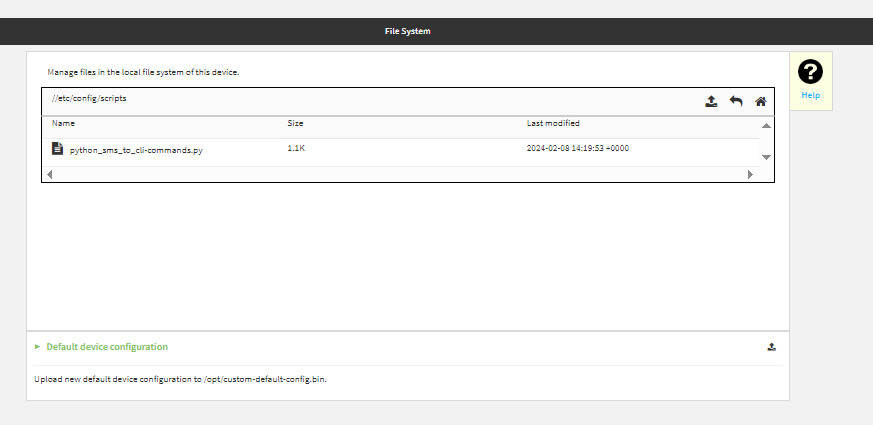
5. Once the script is on the filesystem, you can configure it to run every minute, going to System > device configuration>system>scheduled task>custom scripts>add script (be sure to select the “Allow Scheduled scripts to handle SMS” option and deselect the “Sandbox” option):
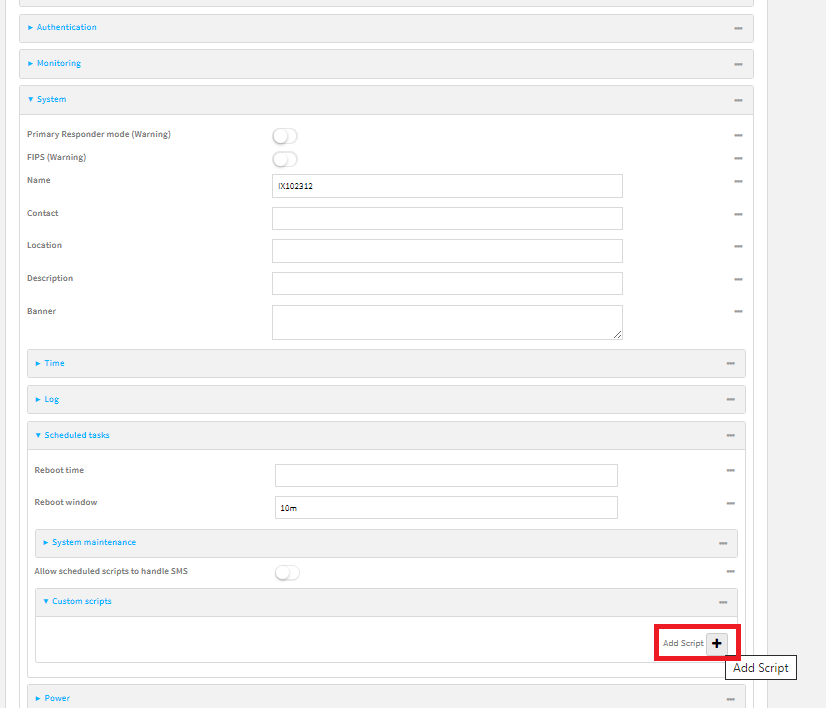
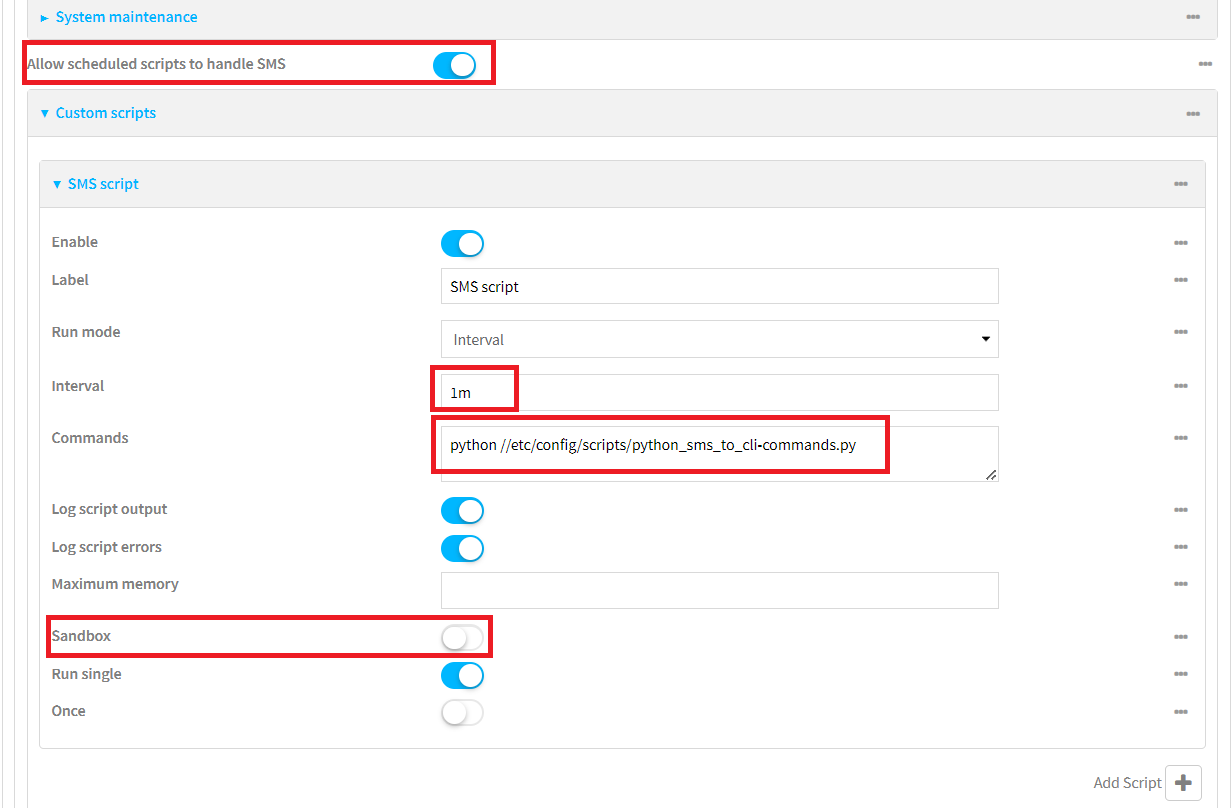
Note: the script itself will listen for incoming SMS messages for a minute and then exit either once a SMS message is received or if 60 seconds elapse, so have it configured each minute ensures that the system keeps checking for incoming SMS messages and process them to perform any CLI commands dictated by the received SMS message
After the SMS is received, the CLI command is run and the following can happen:
- If the CLI command being run has output, it will send that output as a response SMS message.
- If the CLI command being run has no output but ran successfully, the script will instead send an OK response SMS message.
- If the CLI command did not run successfully and have error messages as output, those error messages will sent as a SMS response.
The following can also help when using this script/SMS procedure:
Last updated:
Feb 20, 2024